What is a Prefab in Unity?
When you play a game with an endless number of the same enemies attacking you, how do you think that works? Did the developer make each of those enemies separately? Or did they use something like a prefab in Unity?
A prefab is a specific GameObject that can be reused as many times as needed in a game without having to make that GameObject from scratch each time. It is a template to create new instances of that GameObject.
Prefabs are used very much in Unity. They are used as projectiles, enemies, obstacles, powerups, and so much more. They are basically used for anything you need in the scene that will be used or instantiated more than once. They are very useful to understand and use in your game.
How do I Create a Prefab in Unity?
It is very easy to create a prefab. Drag the GameObject you want to make a Prefab from the Hierarchy Window down into the Project Window. You will see the text color change to a light blue in the Hierarchy Window. To keep things organized, you should create a Prefabs folder in the Assets folder of the Project Window and store your Prefabs there.
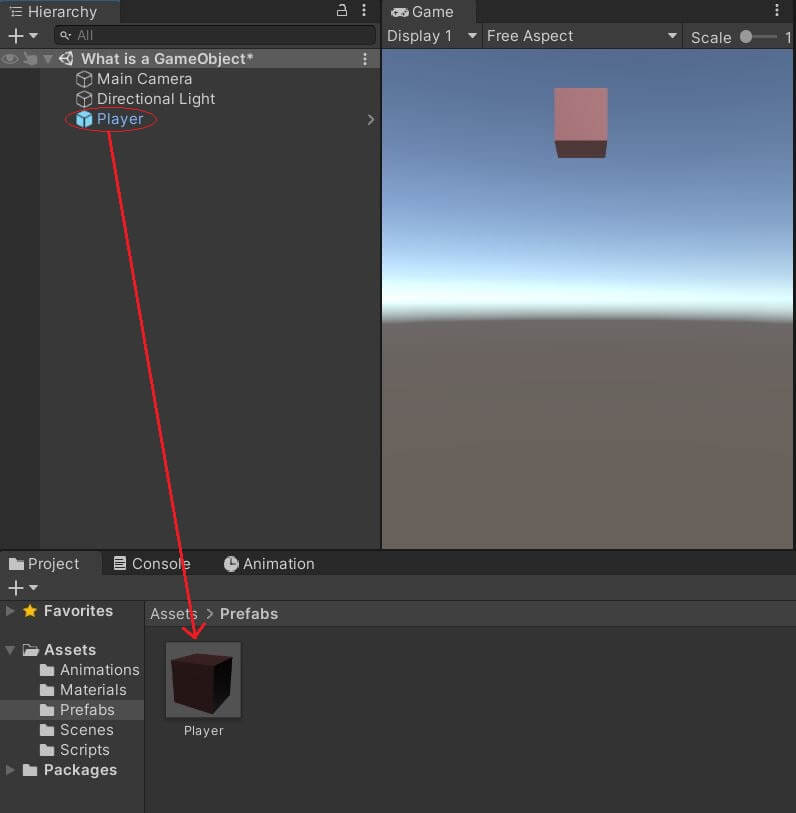
How do I Use a Prefab in Unity?
You can use your Prefabs just like you use any other GameObject. Adding it to the Hierarchy, will add it to the Scene. You can also have your Prefab instantiated while your app is running. This is very useful for actions that will use that specific Prefab many times while your app is running.
Below is a very simple example of how you could use a the Instantiate() function to spawn the same Prefab when you press or hold the space bar on the keyboard.
First, create a GameObject. I called it Spawner. This can be empty or have a mesh filter and mesh render. It does not matter for this example. It will only be used to instantiate the Prefab. Also, create a new C# script, and attach it to that GameObject. Below is the code that will be in the script.
using UnityEngine;
public class InstantiatePrefab : MonoBehaviour
{
public GameObject projectile;
// Update is called once per frame
void Update()
{
if (Input.GetKey("space"))
{
Instantiate(projectile);
}
}
}
We will go through each line of code that is important to this example.
public GameObject projectile;- This line declares a public container named “projectile” to hold a GameObject. We will make the GameObject it will hold soon.
if (Input.GetKey(“space”))- This if statement is checking if the user has pressed or is holding the space bar on the keyboard. If the user is, then the code within the if() statement’s brackets will execute.
Instantiate(projectile);- Finally, we call the Instantiate() function to create an instance of our projectile GameObject in our scene when the space bar is pressed. Instantiate()’s parameter will be the projectile container you declared at the beginning of the script.
Make sure the script is saved, and go back to the Unity Editor.
Next create another GameObject. In this case, I created a capsule and gave it a green Material for the color and named it Bullet. I also added a RigidBody to it so that it can interact with physics when it is instantiated. You do not have to do this. It depends on the needs of your game.
Drag that GameObject from the Hierarchy Window down into the Project Window. You just made a Prefab! It is a very simple process.
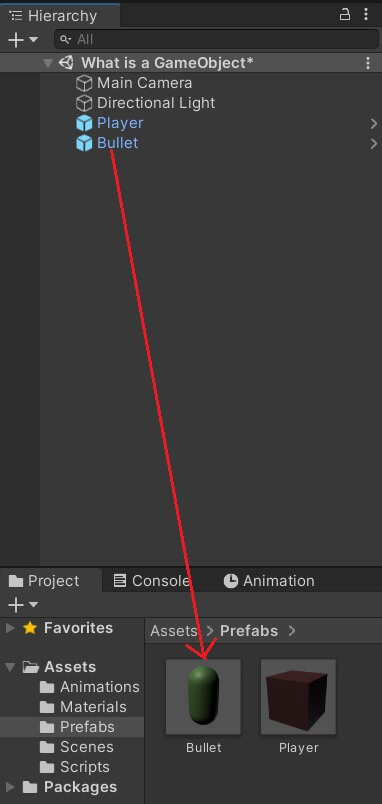
Now that you have the Prefab made, select the first GameObject that has the script attached to it, and look at the script component in the Inspector. You will see a field named “Projectile” (in my example) and a selector box to add a GameObject to it. As the selector shows, it only takes a GameObject. You cannot put another type of object in that box. It must be a GameObject.
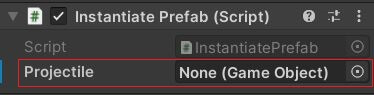
Finally, drag your Prefab GameObject from teh Project Window into the original GameObject’s script in the Inspector Window. Drag it into the box that holds the GameObject. This allows the script to know what GameObject needs to be used and instantiated in our example.
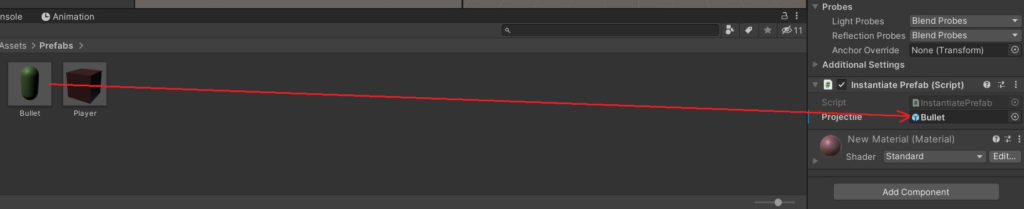
So if you were to run your game and press the space bar, it would look something like this:
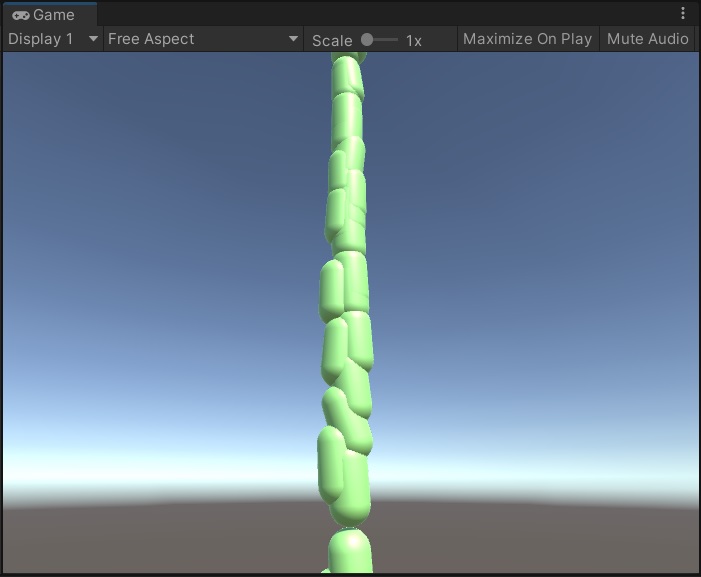
As you can see, many Bullet Prefabs were created after tapping the space bar. As was mentioned before, Prefabs can be used for many gameplay elements – bullets in a gun, powerups that appear for a few seconds, then disappear, a continuous spawn of enemies in a survival mode, and much, much more. Generally, most objects in a game will be Prefabs.
What is the Difference Between a Prefab and GameObject in Unity?
So is there really a difference between a GameObject and a Prefab? Yes and no. A Prefab is a GameObject, however, it allows you to make an exact duplicate of a GameObject in a Game that allows for the use of that same premade GameObject as many times as you want to be used in different aspects of gameplay.
For example, in this tutorial, if you would’ve just created a GameObject and dragged it from the Hierarchy Window into the Spawner’s script, as we did with our Bullet Prefab, a capsule would have instantiated into the scene, but it would not have any custom characteristics or attributes need to be, for example, a bullet, or an enemy. It would just be a simple capsule.
You could also choose to use a customized GameObject you made and just duplicate it yourself in your scene. But for example, if you needed to change its appearance or make it move a different way, you would have to change it one-by-one on each copy of the GameObject in your game. With a Prefab, you can open the Prefab Mode.
In order to enter Prefab Mode, do one of the following:
- Double-click on the Prefab in the Project Window, or
- Select the Prefab in the Project Window. Then click on the “Open Prefab” button in the Inspector Window.
Prefab Mode is shown below. It’s the same look of the Unity Editor, but it only shows information for the one Prefab you selected. Here you can edit the Prefab however you like, save it, and then all of the instances of that Prefab already in the game will reflect those edits.
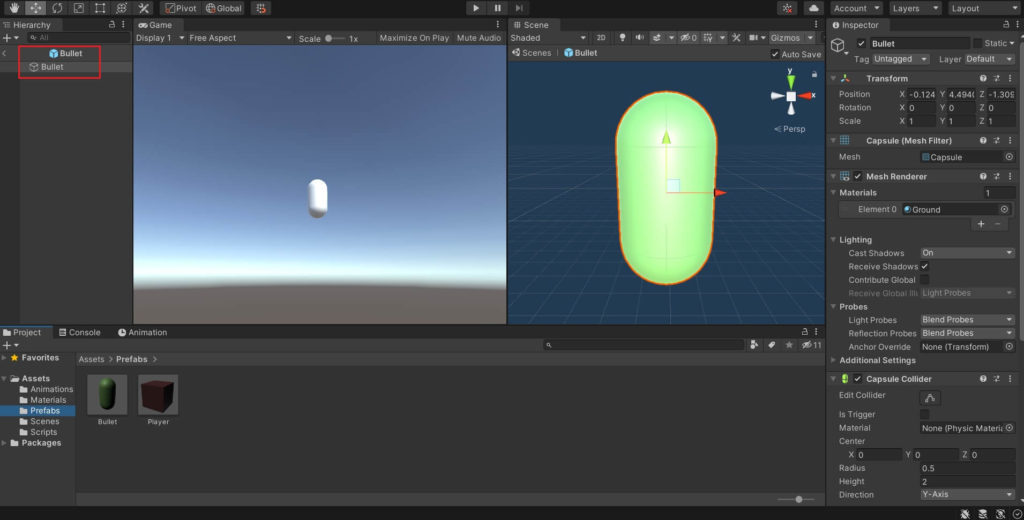
Should I Use Prefabs in Unity?
The short answer is absolutely. Any GameObject you plan on using more than once in your game should be a Prefab. Weapons, powerups, particle systems, and so much more. Prefabs provide an easy way to reuse and edit a GameObject multiple times without having to change each instance of the GameObject one at a time.
Would you like to learn how to fire a projectile using a Prefab? Click here to find out how!